Get
Fetch data from your database quickly and securely.
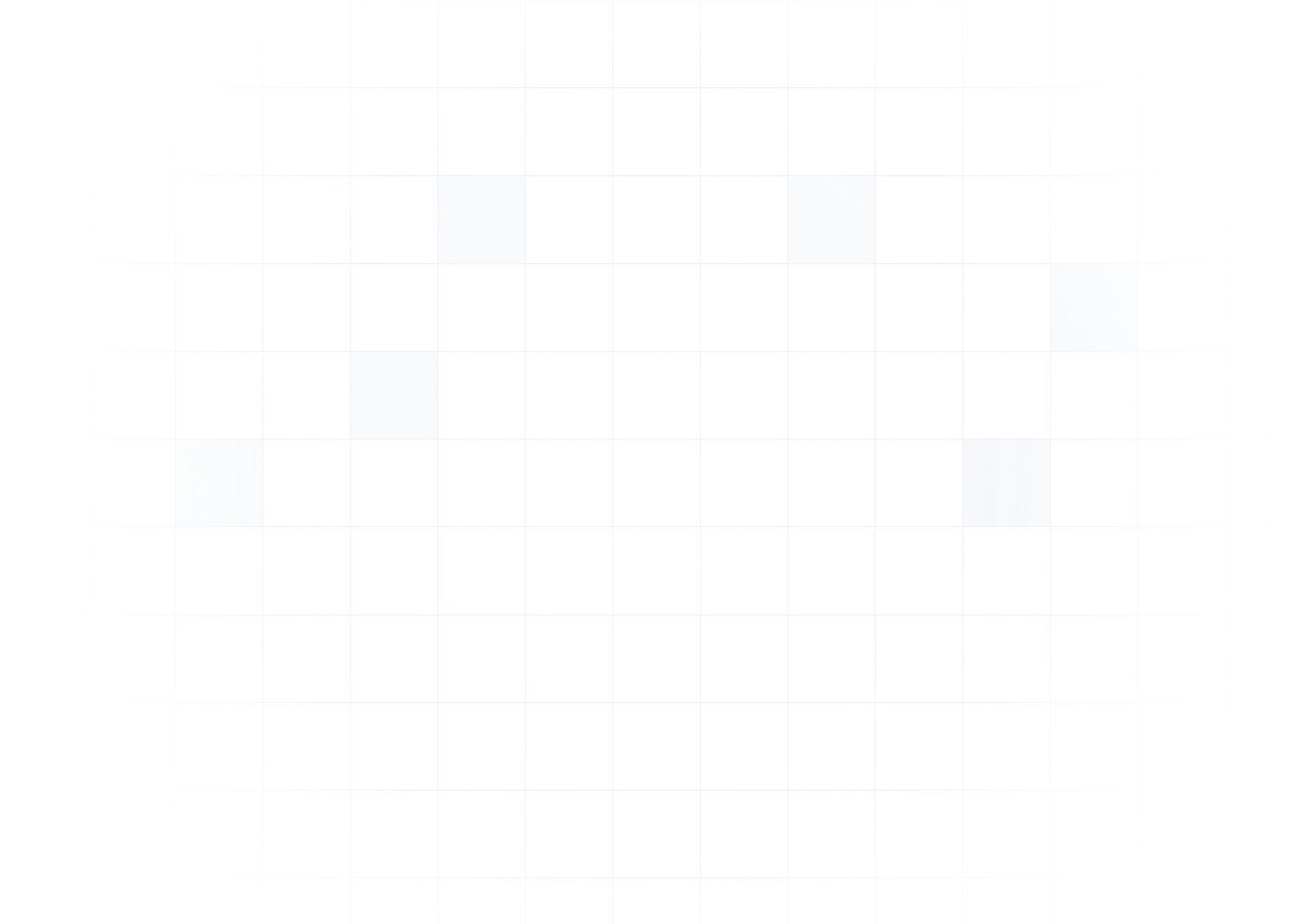
Overview
The get method only retrieves data. It cannot be used to write or update data. It was created to be the fastest way to access your data, that explains the key length. You can get data using the npm module or via any HTTP request.
Using CURL
CURL https://datasquirel.com/api/query/get?db=db_name&query=SELECT+*+FROM+table_name -H "Authorization:READ_ONLY_API_KEY";Content-Type:application/json
Using node module
const datasquirel = require("datasquirel"); datasquirel .get({ db: "test", key: process.env.DATASQUIREL_READ_ONLY_KEY, query: "SELECT title, slug, body FROM blog_posts", }) .then((response) => { console.log(response); });
The response
The response from this method returns a JSON payload with two fields: success: which could be either true
or false
, and payload: which is an array of values when the operation is successfull, or anything from null
to a string to an object with an error message. This is a sample of a successful response object:
{ success: true, payload: [ { id: 1, title: "Hello World", slug: "hello-world", body: "This is a test blog post.", }, ], }
A failed response could return any of three results:
- A
null
payload.{ success: false, payload: null }
- An error string payload
{ success: false, payload: "ERROR: no such table as 'blog_posts'" }
- An object payload containing an error field
{ success: false, payload: { error: "MYSQL ERROR: syntax error in your sql" } }
In each case, the success key refurns a value of false
, meaning the query failed.