Post
Full CRUD operations on your database using our feature-rich API integration.
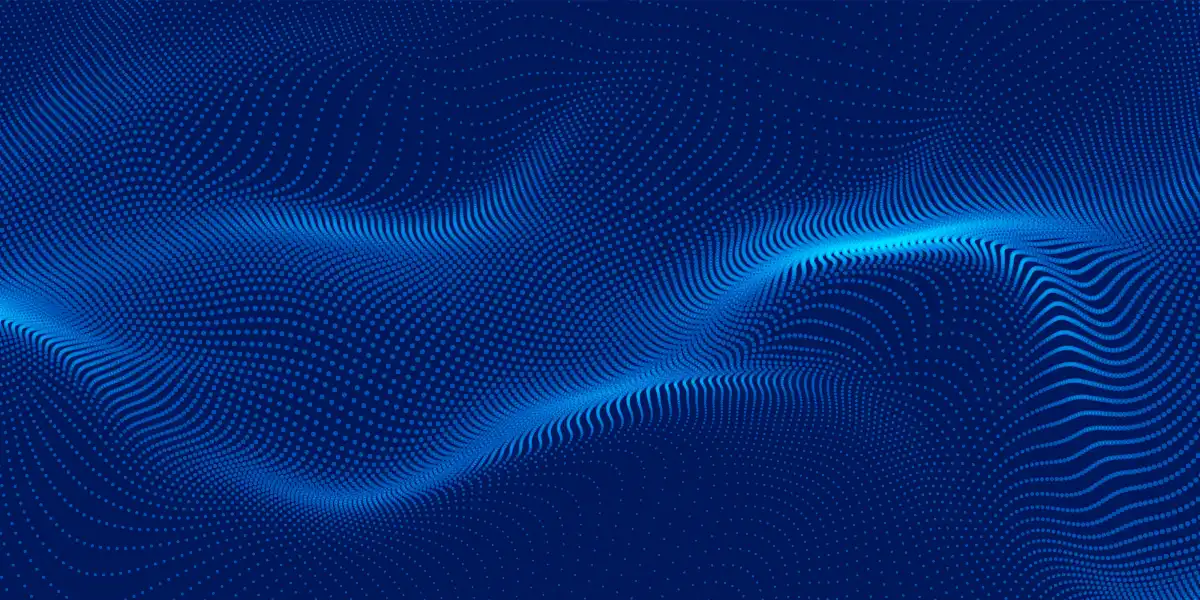
Overview
The post
method expands on the get
method. It adds the ability to insert, update, and delete data, as well as add and delete images from your static files directories.
The post method contains the full spectrum of CRUD operations. And it works *only with the Full Access API key. The read only API key will not work for post methods.
Using CURL
CURL --json '{ "database": "social_network", "query": "UPDATE users SET name = 'John' WHERE id = 1" }' https://datasquirel.com/api/query/post -H "Authorization:FULL_ACCESS_API_KEY"`
Using our npm module
const datasquirel = require("datasquirel"); datasquirel .post({ database: "social_network", key: process.env.FULL_ACCESS_API_KEY, query: "UPDATE users SET name = 'John' WHERE id = 1", }) .then((response) => { console.log(response); });
The Response
The process yeilds simalar results, but with a slight difference: for operations like insert and update, the success
field yeilds true
while the payload
field yeilds an object containing fields like
{ success: true, payload: { serverStatus: 37, affectedRows: 1, } }
Post with object query
The post method can also take an object as the query
instead of a string. Example:
const datasquirel = require("datasquirel"); datasquirel .post({ database: "social_network", key: process.env.FULL_ACCESS_API_KEY, query: { action: "update", table: "users", data: { name: "John", }, identifierColumnName: "id", identifierValue: 1, }, }) .then((response) => { console.log(response); });
This yields the exact same result as before.
Adding media
In addition to the full CRUD operations the post
method offers, you can also add media to your static files directory. This uses the same post method, but with sligtly different parameters. Also, the media you send *must be in base64
format. You can use our npm client module to convert images and documents to base64
format.
Using our node module
const datasquirel = require("datasquirel"); datasquirel.media .uploadImage({ key: process.env.DATASQUIREL_FULL_ACCESS_API_KEY, payload: { imageData: "--------- LONG BASE64 STRING ---------", imageName: "sunflower", folder: "flowers", // Optional mimeType: "jpeg", // Optional thumbnailSize: 300, // Optional }, }) .then((response) => { console.log(response); });